Note
Go to the end to download the full example code.
Handling errors#
By default, pyquickbench.run_benchmark()
will try to benchmark as much as possible even if the callables to be benchmarked throw errors. These errors are caught and the corresponding value in the benchmark is recorded as np.nan
, which will in turn show in plots as a missing value.
import pyquickbench
def comprehension(n):
if n == 1 :
raise ValueError('Forbidden value')
return ['' for _ in range(n)]
def star_operator(n):
if n == 8:
raise ValueError('Forbidden value')
return ['']*n
def for_loop_append(n):
if n == 32:
raise ValueError('Forbidden value')
l = []
for _ in range(n):
l.append('')
all_funs = [
comprehension ,
star_operator ,
for_loop_append ,
]
n_bench = 8
all_sizes = np.array([2**n for n in range(n_bench)])
pyquickbench.run_benchmark(
all_sizes ,
all_funs ,
show = True ,
)
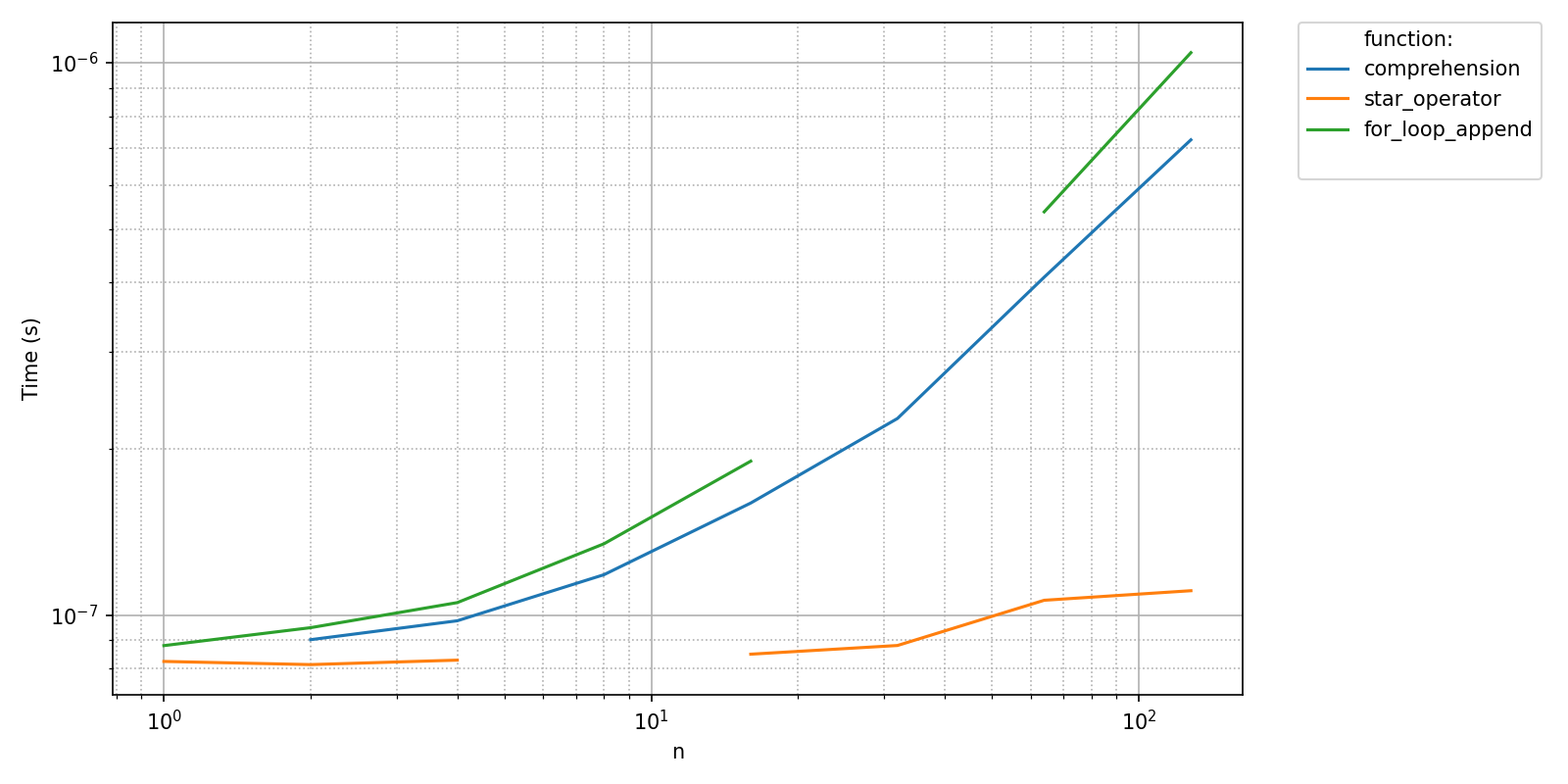
This default can be overriden with the argument StopOnExcept
set to True
. The error is then not caught by pyquickbench.run_benchmark()
, and it is the user’s responsibility to handle it.
Exception thrown: Forbidden value