Note
Go to the end to download the full example code.
Preparing inputs#
By default, the functions to be benchmarked with pyquickbench.run_benchmark()
are expected to take as arguments a single integer n
as in the following code.
def comprehension(n):
return ['' for _ in range(n)]
def star_operator(n):
return ['']*n
def for_loop_append(n):
l = []
for _ in range(n):
l.append('')
all_funs = [
comprehension ,
star_operator ,
for_loop_append ,
]
n_bench = 12
all_sizes = [2**n for n in range(n_bench)]
pyquickbench.run_benchmark(
all_sizes ,
all_funs ,
show = True ,
)
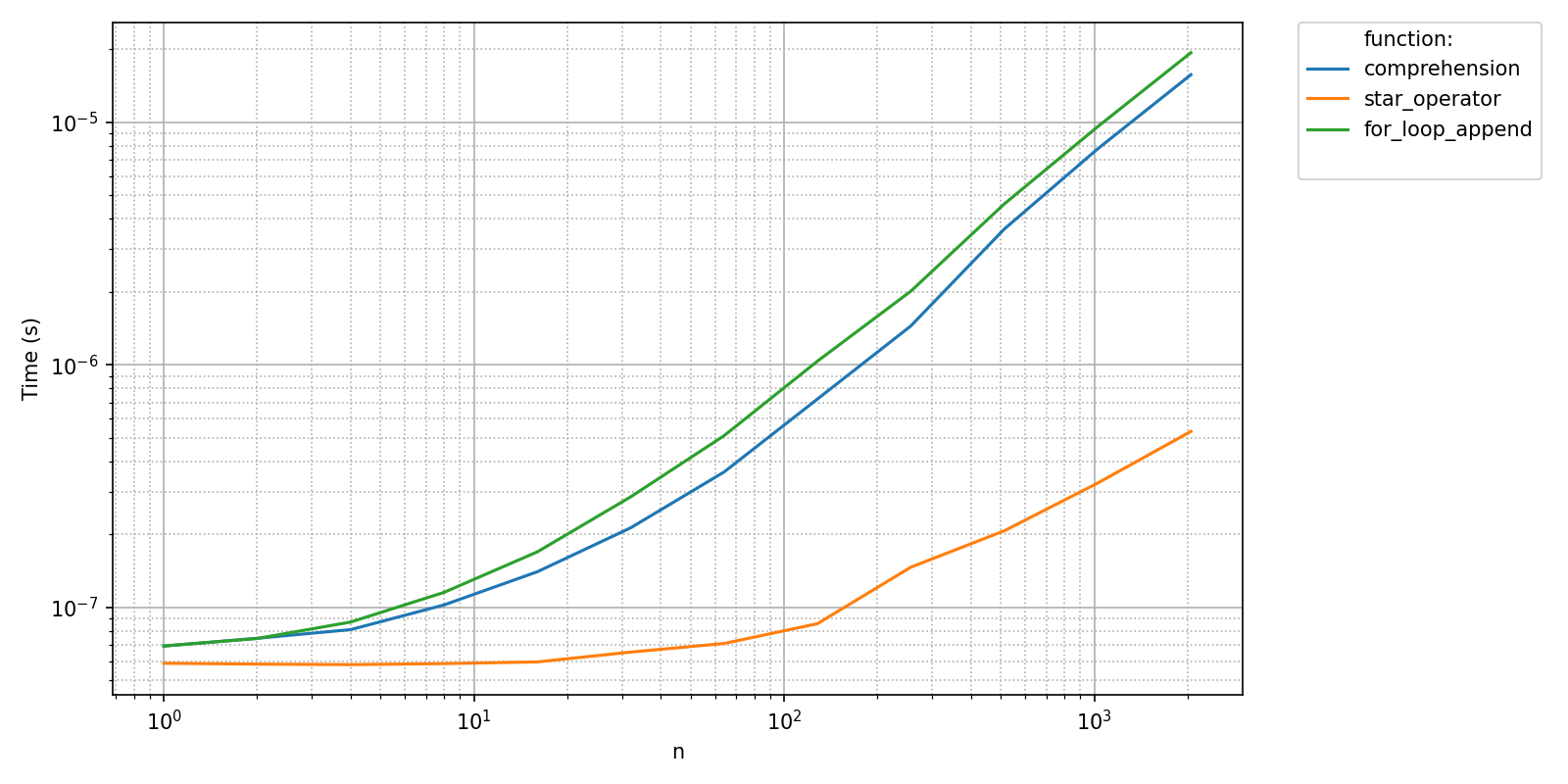
Most often however, the functions to be benchmarked take data as input that is more complex than a simple integer and a setup phase is needed. In the following example, we want to compare different implementations of array summation algorithms.
Here, we want the benchmark to measure the time taken by the different implementations as a function of the size of the input. The setup
argument expects a callable that provides arguments for our different implementations to be timed. For instance, setup
can return a dictionnnary kwargs
, so that each function to be benchmarked will be called as fun(**kwargs)
.
def setup(n):
x = np.random.random(n)
return {'x' : x}
pyquickbench.run_benchmark(
all_sizes ,
all_funs ,
setup = setup ,
show = True ,
)
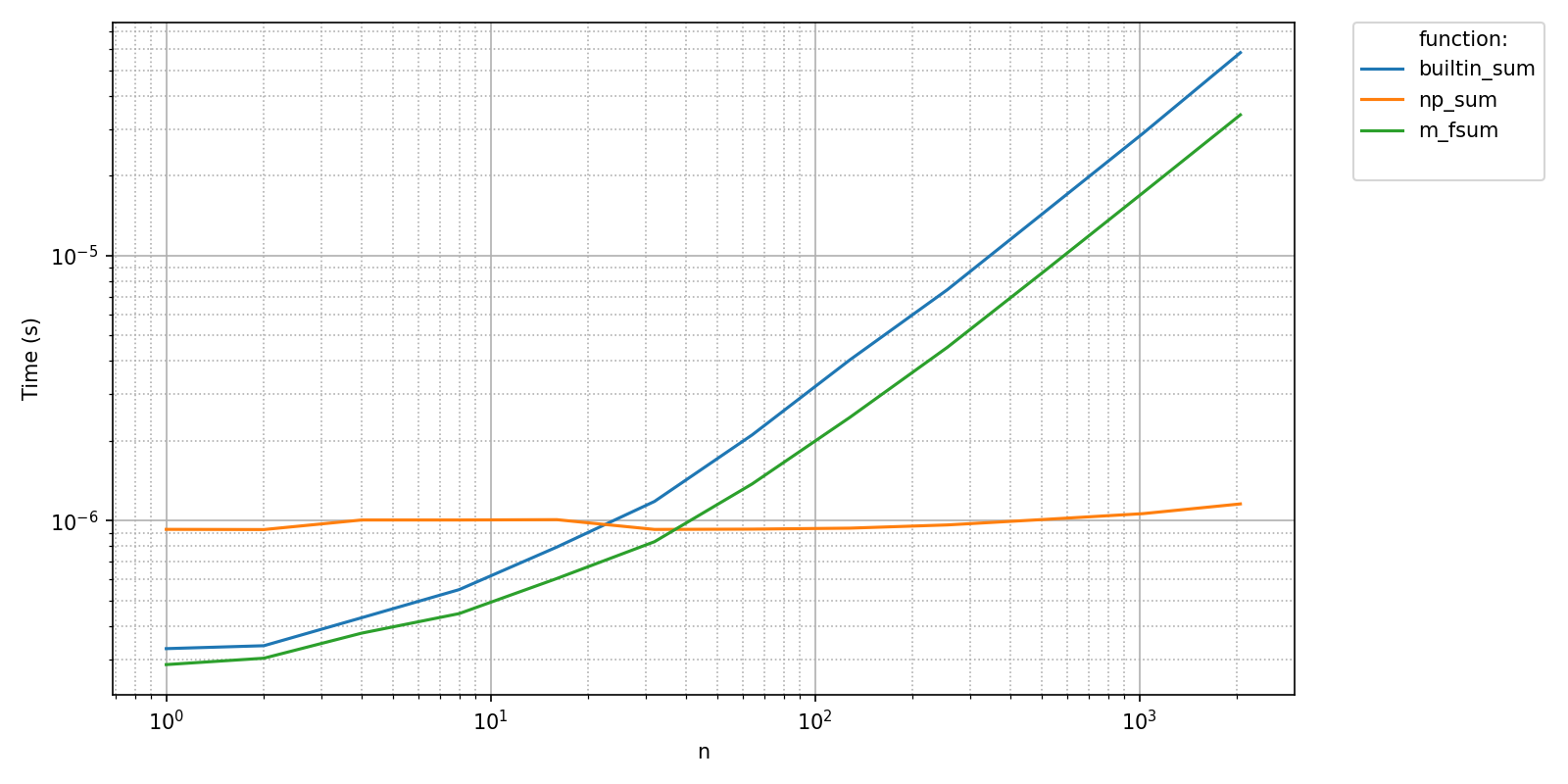
This calling convention is why positional-only arguments are disallowed in pyquickbench.run_benchmark()
. For instance, even though the following defines a legal Python 3.8+ function
def pos_only_fun(n,/):
return n
print(pos_only_fun(42))
42
It is not allowed in pyquickbench.run_benchmark()
since the following raises an error:
try:
print(pos_only_fun(n=42))
except TypeError as err:
print(f'TypeError: {err}')
TypeError: pos_only_fun() got some positional-only arguments passed as keyword arguments: 'n'
This comes with hardly any loss of generality since it is possible to wrap these positional-only arguments functions.
def wrap_fun(n):
return pos_only_fun(n)
print(wrap_fun(42))
print(wrap_fun(n=42))
42
42