Note
Go to the end to download the full example code.
A first benchmark#
Let’s run and plot a first simple benchmark in Python.
Suppose I want to compare the efficiency of a few different methods to pre-allocate memory for a list of strings in Python.
Let’s define a separate python function for three different list pre-allocation strategies.
These functions all take an integer called n
as an input, which stands for the length of the list to be pre-allocated.
The argument name n
is the pyquickbench.default_ax_name
and can be changed in as described in Preparing inputs.
def comprehension(n):
return ['' for _ in range(n)]
def star_operator(n):
return ['']*n
def for_loop_append(n):
l = []
for _ in range(n):
l.append('')
all_funs = [
comprehension ,
star_operator ,
for_loop_append ,
]
Let’s define relevant sizes of lists to be timed.
Now, let’s import pyquickbench, run and plot the benchmark
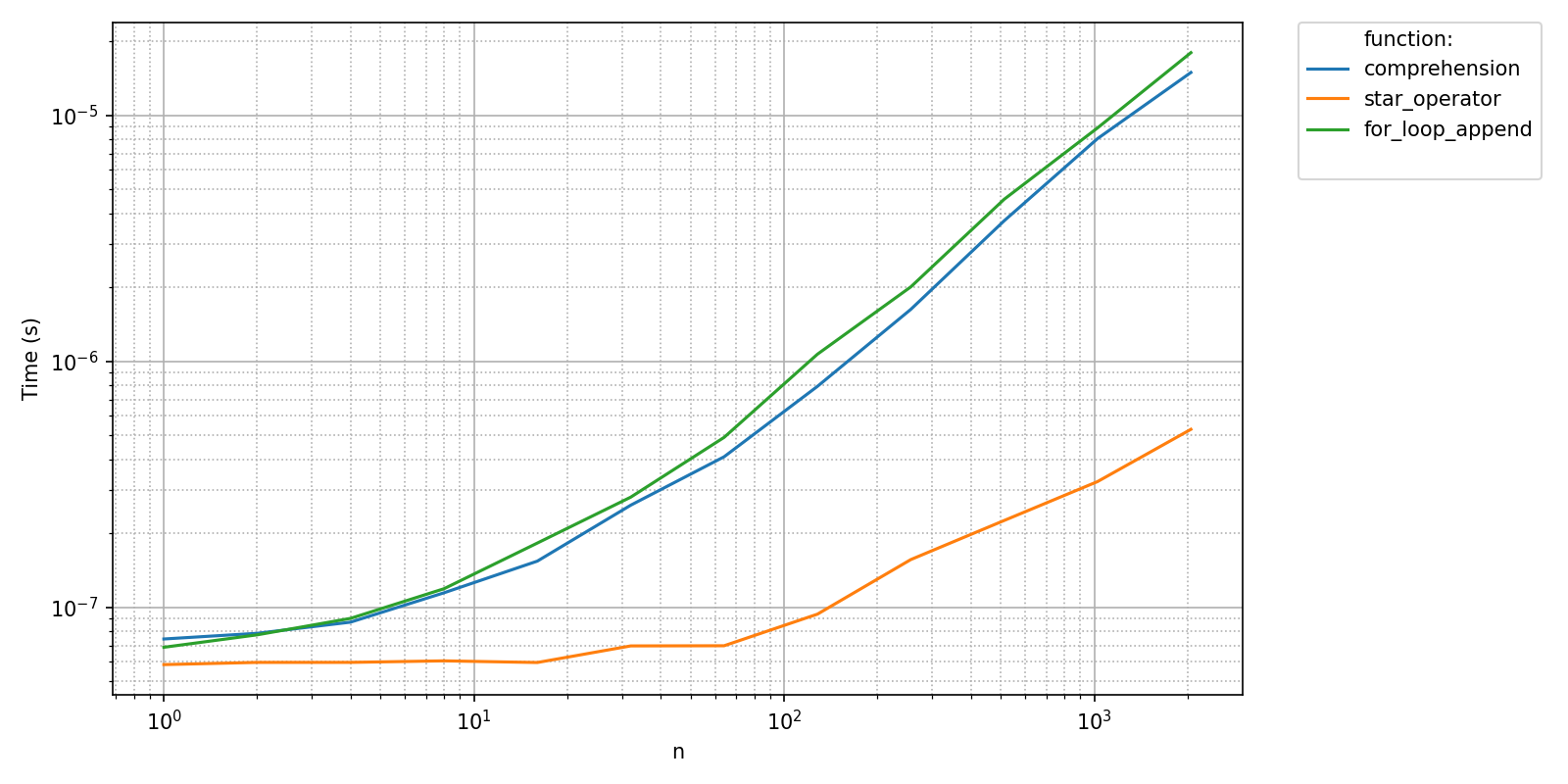
From this benchmark, it is easy to see that initializing a list to a given length filled with a single value is quickest using the star operator.