Note
Go to the end to download the full example code.
Numpy array initialization#
This benchmark compares the execution time of several numpy.ndarray
initialization routines.
import numpy as np
import pyquickbench
dtypes_dict = {
"float32" : np.float32,
"float64" : np.float64,
}
def zeros(n, real_dtype):
np.zeros((n), dtype=dtypes_dict[real_dtype])
def ones(n, real_dtype):
np.ones((n), dtype=dtypes_dict[real_dtype])
def empty(n, real_dtype):
np.empty((n), dtype=dtypes_dict[real_dtype])
def full(n, real_dtype):
np.full((n), 0., dtype=dtypes_dict[real_dtype])
all_funs = [
zeros ,
ones ,
empty ,
full ,
]
all_args = {
"n" : np.array([2**n for n in range(0, 30)]),
"real_dtype" : ["float32", "float64"],
}
def setup(n, real_dtype):
return {'n': n, 'real_dtype':real_dtype}
all_timings = pyquickbench.run_benchmark(
all_args ,
all_funs ,
setup = setup ,
filename = filename ,
StopOnExcept = True ,
ShowProgress = True ,
)
plot_intent = {
"n" : 'points' ,
"real_dtype" : 'curve_linestyle' ,
pyquickbench.fun_ax_name : 'curve_color' ,
}
pyquickbench.plot_benchmark(
all_timings ,
all_args ,
all_funs ,
plot_intent = plot_intent ,
show = True ,
)
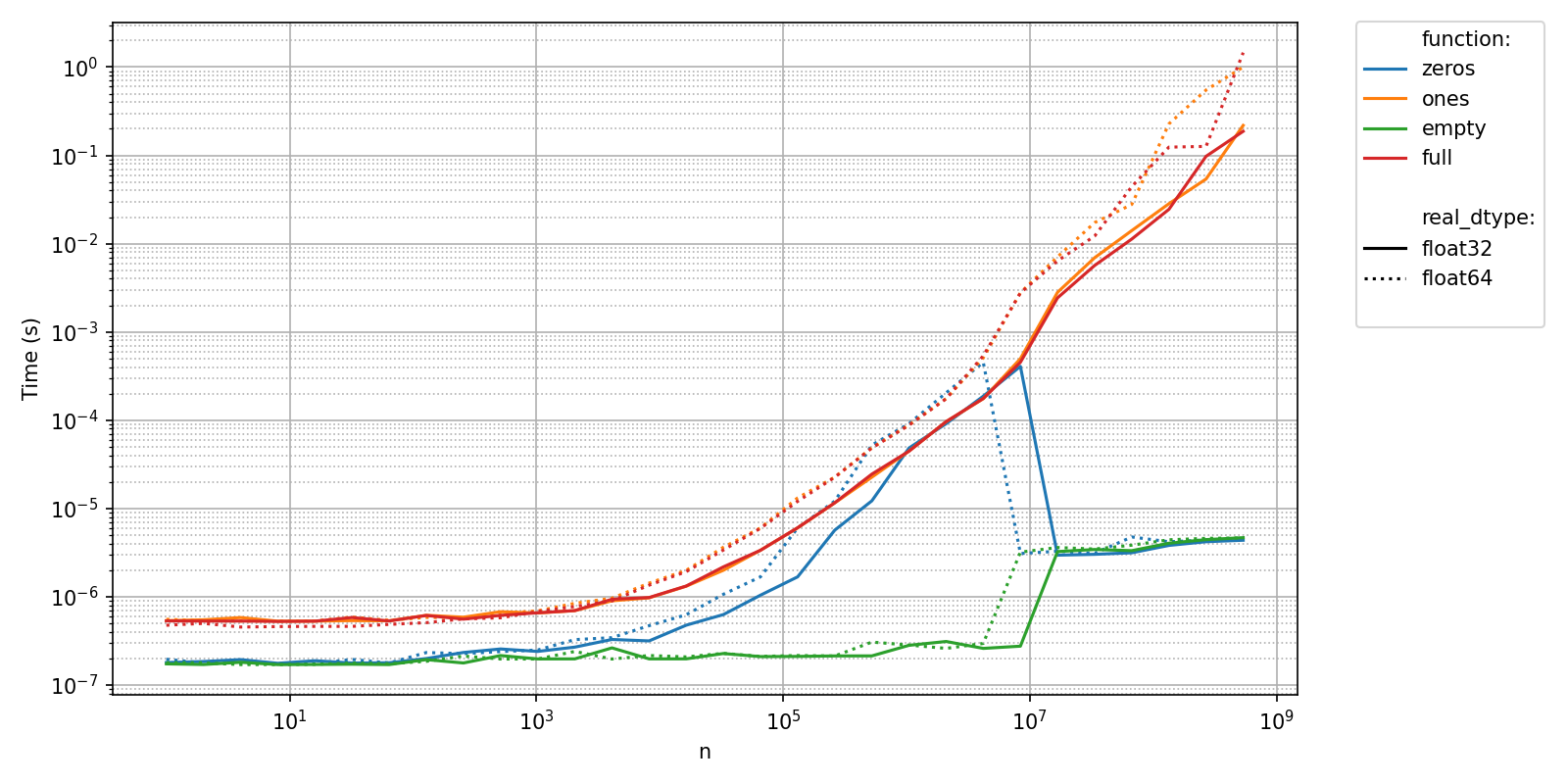
While these measurement seem surprizing, they are explained in the numpy documentation :
Be mindful that large arrays created with
np.empty
ornp.zeros
might not be allocated in physical memory until the memory is accessed. […] One can force pagefaults to occur in the setup phase either by callingnp.ones
orarr.fill(value)
after creating the array.