Note
Go to the end to download the full example code
Convergence analysis of integration methods on segment#
Evaluation of relative quadrature error with the following parameters:
fun_names = [
"exp",
]
methods = [
'Gauss',
'Radau_I',
'Radau_II',
'Lobatto_III',
]
# all_nsteps = range(2,11)
all_nsteps = [5]
refinement_lvl = np.array(range(1,100))
# refinement_lvl = np.array([2**i for i in range(18)])
The following plots give the measured relative error as a function of the number of quadrature subintervals
plt.show()
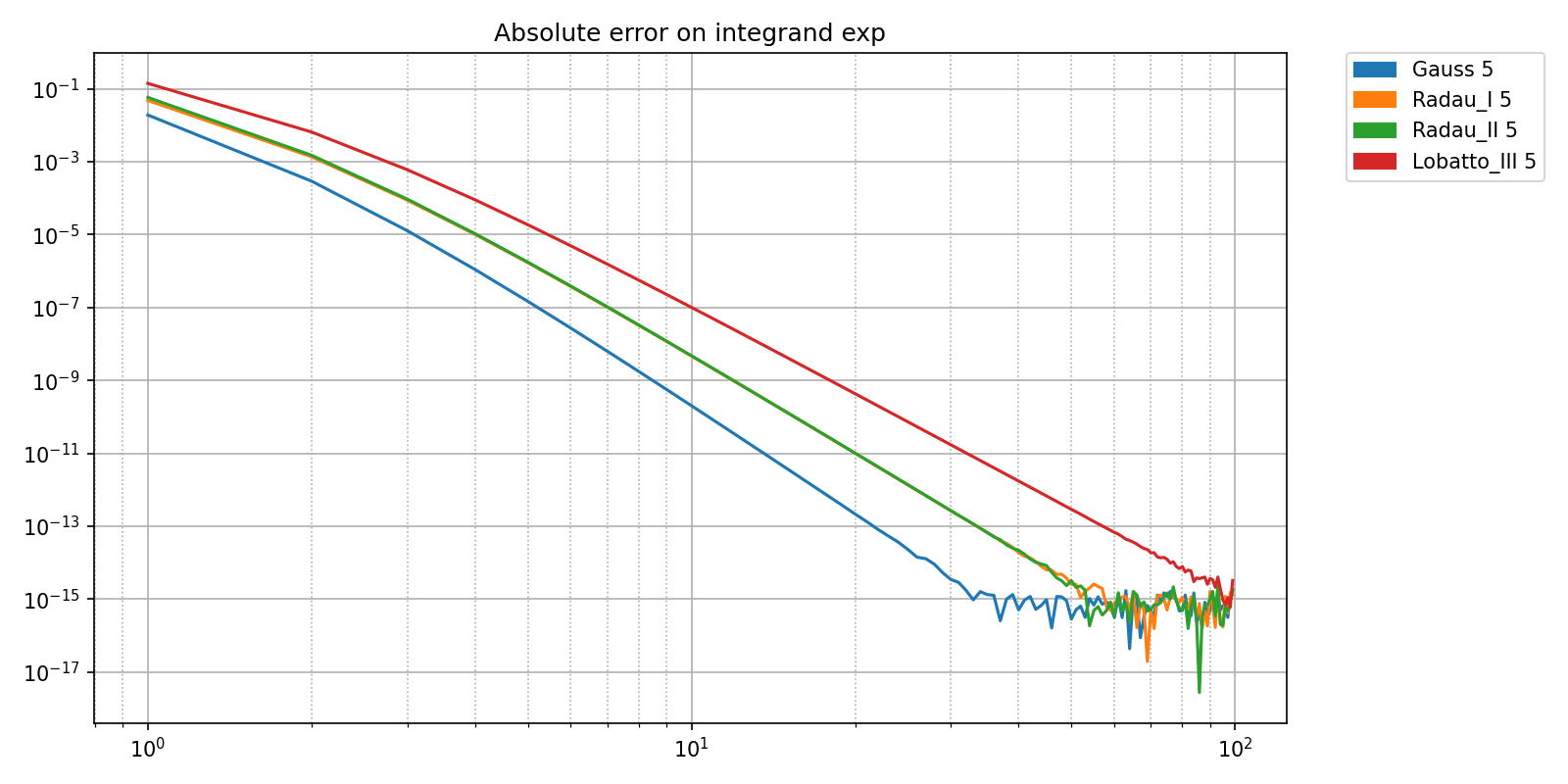
The following plots give the measured convergence rate as a function of the number of quadrature subintervals. The dotted lines are theoretical convergence rates.
plt.show()
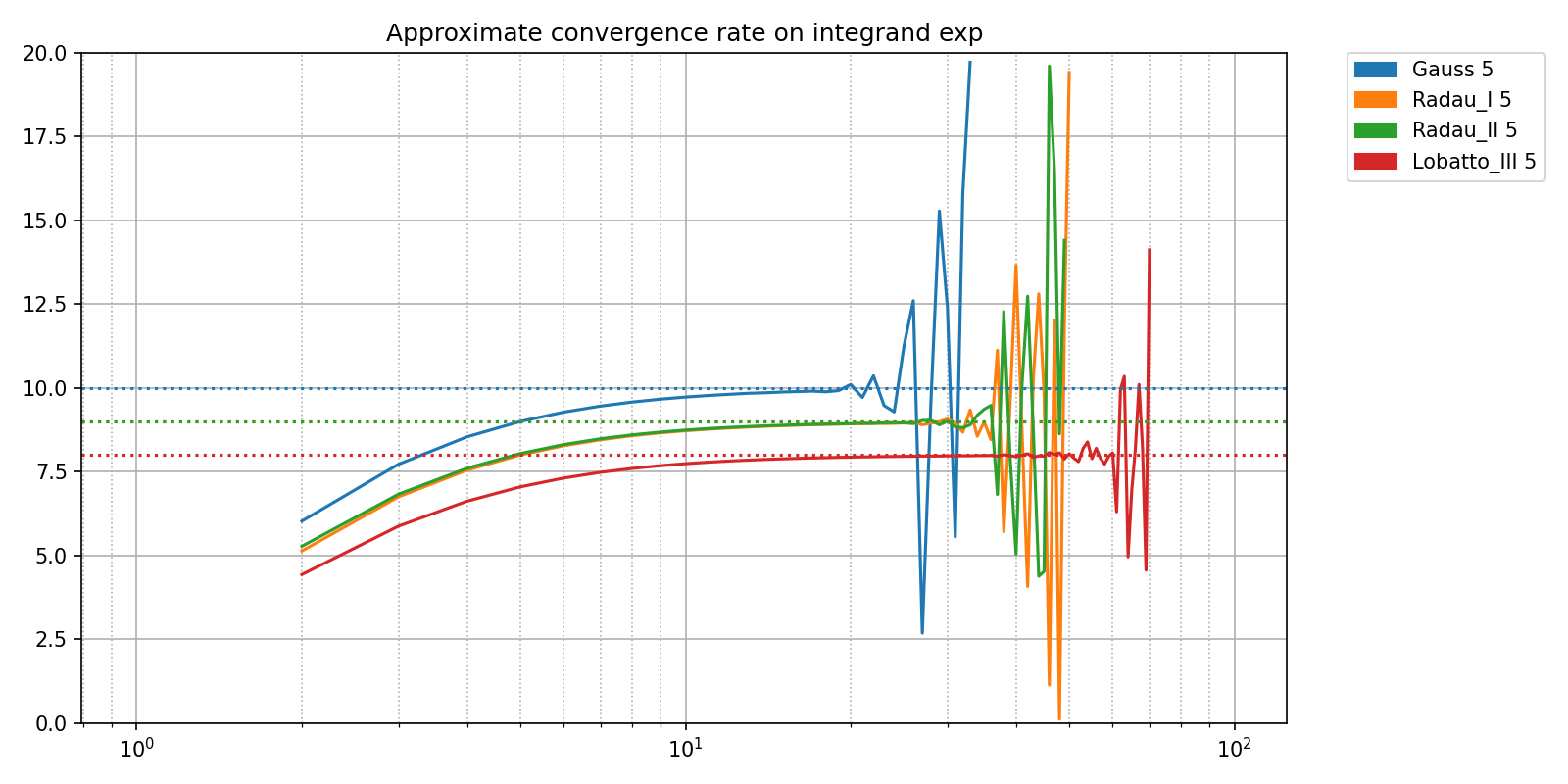
We can see 3 distinct phases on these plots:
A first pre-convergence phase, where the convergence rate is growing towards its theoretical value. the end of the pre-convergence phase occurs for a number of sub-intervals roughtly independant of the convergence order of the quadrature method.
A steady convergence phase where the convergence remains close to the theoretical value
A final phase, where the relative error stagnates arround 1e-15. The value of the integral is computed with maximal accuracy given floating point precision. The approximation of the convergence rate is dominated by seemingly random floating point errors.
Total running time of the script: (0 minutes 0.666 seconds)